반응형
바닐라 JS로 메타마스크 연결 및 이더리움 송금 - vscode Live server에서 실행
<!DOCTYPE html>
<html>
<head>
<title>Page Title</title>
<meta charset="utf-8">
<script>
let accounts;
window.onload = function(){
// 지갑 변경 시 handleAccountsChanged 함수 호출,
if (window.ethereum){
this.ethereum.on('accountsChanged', handleAccountsChanged);
window.ethereum.request({ method: 'eth_accounts'})
.then(handleAccountsChanged)
.catch((err) => {});
} else { alert("Please install metamask app"); }
}
const handleAccountsChanged = (acc) => {
// 변경된 지갑 주소
accounts = acc;
// 지갑이 연결된 상태인 경우
if ( accounts && accounts.length > 0 )
{
// connectwallet 버튼 숨김
} else {
}
}
// Metamask 연결 요청
const enableEth = async () => {
accounts = await window.ethereum.request({ method: 'eth_requestAccounts'})
.catch((err)=>{ console.log(err.code); });
console.log( accounts );
}
// 연결된 계정의 잔액 조회
const checkEthBalance = async () => {
let balance = await window.ethereum.request({ method: 'eth_getBalance',
params: [ accounts[0], 'latest' ]
}).catch((err) => {
console.log(err);
});
balance = parseInt(balance);
balance = balance / Math.pow(10,18);
console.log(balance);
}
// 다른 계정으로 이더리움 전송
const sendTransaction = async () => {
const PRICE = 0.12; // ETH
const ADDRESS = '0x74b55Ab6Da3BB6420F5cf80273F22d4ace4045Bc';
const ETH_GAS = 65;
let params = [
{
from: accounts[0],
to: ADDRESS,
gas: Number(21000).toString(16),
gasPrice: (GAS_FEE * 10 ** 9).toString(16),
value: (PRICE * 10 ** 18).toString(16)
}
]
let result = await window.ethereum.request( {method: 'eth_sendTransaction', params } ).catch((err) => {
console.log(err);
});
}
</script>
</head>
<body>
<button onclick="enableEth()">Connect Wallet</button>
<button onclick="checkEthBalance()">Check my balance</button>
<button onclick="sendTransaction()">Send transaction</button>
</body>
</html>
<!DOCTYPE html>
<html>
<head>
<title>Page Title</title>
<meta charset="utf-8">
<script src="main.js"></script>
</head>
<body>
<button onclick="enableEth()">Connect Wallet</button>
<button onclick="checkEthBalance()">Check my balance</button>
<button onclick="sendTransaction()">Send transaction</button>
</body>
</html>
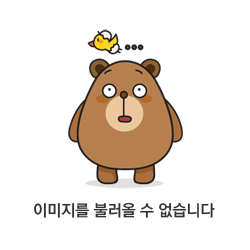
react 메타마스크 지갑 연결 및 스마트컨트랙트
- 프로젝트 생성
> npx create-react-app SimpleStore
> cd SimpleStore
> npm install ethers
> npm start
import React, {useState} from 'react'
import {ethers} from 'ethers'
import SimpleStore_abi from './SimpleStorage_abi.json'
const SimpleStore = () => {
// in remix, ENVIROMENT Web3 Provider, deploy address
const contractAddress = '0xd9145CCE52D386f254917e481eB44e9943F39138';
const [errorMessage, setErrorMessage] = useState(null);
const [defaultAccount, setdefaultAccount] = useState(null);
const [connButtonText, setConnButtonText] = useState('Connect Wallet');
const [CurrentContractVal, setCurrentContractVal] = useState(null);
const [provider, setProvider] = useState(null);
const [singer, setSinger] = useState(null);
const [contract, setContract] = useState(null);
const connectWalletHandler = () => {
if (window.ethereum){
window.ethereum.request( { method: 'eth_requestAccounts'})
.then(result => {
accountChangedHandler(result[0]);
setConnButtonText('Wallet Connected');
})
} else {
setErrorMessage('Need to install Metamask!');
}
}
const accountChangedHandler = (newAccount) =>{
setdefaultAccount(newAccount);
updateEtheres();
}
const updateEtheres = () => {
let tempProvider = new ethers.providers.Web3Provider(window.ethereum);
setProvider(tempProvider);
let tempSigner = tempProvider.getSigner();
setSinger(tempSigner);
// smart_contract
let tempContract = new ethers.Contract(contractAddress, SimpleStore_abi, tempSigner);
setContract(tempContract);
}
const getCurrentVal = async() => {
let val = await contract.get();
setCurrentContractVal(val);
}
const setHandler = (event) => {
event.preventDefault();
contract.set(event.target.setText.value);
}
return (
<div>
<h3> {"Get/Set Interaction with contract"} </h3>
<button onClick={connectWalletHandler}>{connButtonText}</button>
<h3> Address: {defaultAccount} </h3>
<form onSubmit={setHandler}>
<input type='text' id='setText'/>
<button type={"submit"}> Update Contract </button>
</form>
<button onClick={getCurrentVal}>Get Current Value</button>
{errorMessage}
</div>
)
}
export default SimpleStore;
반응형
'프로그래밍' 카테고리의 다른 글
파이썬 이미지 RGB 색조 변경 (0) | 2022.03.12 |
---|---|
kaikas API 지갑 연동 (0) | 2022.02.15 |
python exe 파일 만들기 (0) | 2022.01.16 |
안드로이드 계산기 앱 제작 및 배포 (0) | 2021.07.18 |
한국어 문장 생성 AI - KoGPT2 (0) | 2021.06.19 |